Update #5 Rogue Class, Traits, Plates & More!
Welcome to my Devlog!
It’s been a busy stretch of development lately! I’ve been focused on tightening up core systems like player health, death and respawn logic, traps, inventory syncing, and a functional action bar UI. Getting the foundation solid has been the goal so I can build on top of it with more mechanics. A big highlight was getting the networked inventory system finally working — players can now loot, move items between containers, and pick up world items with synced visuals and sounds across all clients. The trap disarm trait for Rogues is also functional, along with the first version of pressure plate puzzles and some early work on player death states with respawn logic. Every system is starting to talk to each other — and it’s feeling more like a game than ever.
Character Classes - Rogue Class
For my game’s character class system, I built a modular, flexible setup that uses ScriptableObjects to define each class and their unique traits. Right now, the only implemented class is the Rogue, but the system is already built to support expansion as more classes are added later.
At the core of the system is the CharacterClass
ScriptableObject. This asset holds all the data about a class: its name, description, icon, base stats (like health and stamina), and a list of traits defined in the TraitType
enum. For example, the Rogue class has the DisarmTraps
trait, which allows it to interact with traps in ways other classes can’t. Traits are what drive class-specific gameplay logic — they’re simple to add and easy to check for using the built-in HasTrait()
method.
Each player in the game has a CharacterClassComponent
attached to their GameObject. This component references their selected CharacterClass
and exposes methods like HasTrait()
so other scripts can easily check if a player has a certain ability. This component is also where things like class-specific animations are triggered, such as the Rogue's disarm animation when successfully disabling a trap.
To organize everything, I also created a ClassManager
singleton. It holds references to all the available class ScriptableObjects and provides helper methods like GetClassByName()
for assigning classes via the UI or during character creation.
The actual trap interaction logic uses these components to determine behavior at runtime. For example, when a player enters a trap trigger, the trap checks if that player’s CharacterClassComponent
has the DisarmTraps
trait. If it does, the player gets a chance to disarm the trap instead of triggering it. This makes the Rogue feel unique and introduces class-specific interactions in the world.
Overall, the system is clean and highly modular. I can easily add new traits, new classes, and build out more gameplay mechanics based on this foundation. The Rogue class is just the first step — next up, I’ll be looking into more class abilities and expanding the gameplay around class identity.
Introducing Character Traits - Rogue
Each character in the game can select one trait during character creation — with plans to eventually allow up to three traits per character. Traits are lightweight but powerful modifiers that allow players to customize their playstyle and contribute uniquely to a party. They’re designed to encourage class variety and group synergy, especially in co-op or party-based exploration.
The Rogue is the first class to utilize this system and serves as a perfect example of how traits shape gameplay. For the Rogue, I’ve planned out three distinct traits: Disarm Traps, Sneak, and Lockpicking. Each one provides a different utility: disabling environmental traps, moving stealthily to avoid detection, or unlocking doors and chests that would otherwise be inaccessible.
Eventually players will choose from multiple traits at a time, making that choice impactful and strategic. This allows for multiple builds of the same class and makes team composition more meaningful. For instance, one Rogue might specialize in sneaking past enemies while another takes on the trap disarming role in a dungeon crawl.
The trait system is built for expansion and gives me a flexible way to add class diversity without bloating the core mechanics. It's simple, modular, and lays the groundwork for more advanced systems down the line.
Trait - Disarm
The Disarm Trap trait is currently exclusive to the Rogue class and allows players to bypass and disable traps throughout the environment. As it stands, any Rogue with this trait will automatically avoid triggering traps, making them a valuable asset in navigating hazardous areas. This system may evolve in the future to incorporate stat-based calculations, such as Dexterity influencing trap detection or disarm success rates.
Disarming a trap isn't guaranteed. When a Rogue attempts a disarm, a virtual “trap roll” occurs behind the scenes. If the roll meets or exceeds the trap’s difficulty threshold, the trap is successfully disabled. On failure, the trap activates, dealing damage to any player within range. This adds a bit of tension and risk to the system — it’s not a free pass.
When a disarm attempt is initiated, the Rogue is temporarily frozen in place while a disarming animation plays, accompanied by a unique sound effect to emphasize the interaction. If successful, the trap is permanently disabled for the rest of the session, ensuring safe passage for other players. This trait not only enhances the Rogue’s role as a scout and utility class, but also encourages teamwork and tactical decisions when navigating dangerous dungeons or ruin-filled landscapes.
Introducing the Stamina System – Design Thoughts & Direction
With the health, damage, and regeneration systems now fully implemented, my attention is shifting to the next core stat: Stamina. This system will play a significant role in shaping how players interact with the world — from exploration to combat, stamina will become a key layer in gameplay depth and decision-making.
So, why is stamina important in a game like this? Stamina acts as a natural limiter on player actions, creating moments of risk and choice. It has the potential to both speed up and slow down gameplay depending on how it's used, which makes it a powerful balancing tool. If implemented well, it can introduce tension and pacing in a way that feels rewarding rather than restrictive.
Stamina will primarily be consumed through movement-based actions like jumping, vaulting, or performing heavy physical actions. One key decision I’ve made early on: running will not consume stamina. However, while sprinting, stamina will not regenerate — this creates a trade-off between speed and endurance without punishing mobility. I think this gives a good middle ground between freedom and resource management.
As for regeneration, stamina will recover over time through passive means. I'm still exploring the possibility of adding consumables or traits that can boost stamina recovery for certain builds or classes. I’ll be prototyping multiple approaches to find what feels right for the game’s pace and challenge curve.
This initial implementation is intentionally lean — I want to get a feel for how stamina affects the player’s rhythm before expanding its influence across more systems like climbing, swimming, or special abilities. The goal is to ensure that stamina feels like a meaningful, intuitive mechanic that complements the rest of the core gameplay loop.
Stamina attributes working so far:
Stamina regeneration rate
Stamina drain rate
Stamina jump cost
Stamina UI slider bar
Pressure Plate Science
I’ve implemented a modular pressure plate puzzle system that can be reused throughout the game in a variety of ways. The system supports linking an array of pressure plates to any object — doors, walls, platforms, or other interactive elements. These plates can be configured to trigger in any order or in a specific sequence, offering flexibility in puzzle design.
The mechanic is simple at its core: step on a pressure plate, hear a satisfying click or sound cue, and somewhere in the level, a door opens. If a player or object leaves the plate, the door closes again — adding tension, timing, and teamwork to puzzle-solving.
Not just limited to players, these plates can be activated by movable objects like statues, crates, or barrels — encouraging creative solutions. To guide players visually, each pressure plate and its linked counterpart (door or mechanism) share matching markings or symbols, helping players piece together what goes where.
This system is designed to be lightweight, reusable, and perfect for layering into dungeons and exploration areas. It’s one of those foundational mechanics that will pop up again and again with increasing complexity.
Networked Inventory & External Item Containers
After a lot of iteration and testing, I’ve officially completed the networked inventory system — and it’s working beautifully! Each player now has their own independent inventory, and items can be seamlessly transferred between inventories and external item containers like chests or loot piles.
One of the biggest wins is that item pickups are fully synced across the network. When a player picks up an item, it now disappears for all other players, preventing duplicates or desync issues. Items also play their unique pickup sound effects, giving players immediate feedback and making each item feel rewarding to grab.
Syncing player inventories across the network was no easy feat — there were a ton of edge cases and bugs to squash. But after countless hours of debugging, I’ve finally got a system in place that’s clean, modular, and reliable. Honestly, I feel like I could set up a networked inventory system in my sleep now.
This sets the foundation for looting, trading, and gear systems — and I’m excited to build on top of it from here.
Action bars are here!
Action Bars (aka Hot Bars) are now fully functional! Players can now drag and drop items directly onto the on-screen hot bar for quick and convenient access during gameplay. Each slot is mapped to a key input, making it easy to trigger items like potions, consumables, or weapons with either a keystroke or mouse click.
These bars are currently dedicated to item usage only — once character abilities are implemented, a separate action bar will be added exclusively for those.
Even though these aren’t final UI designs, they’ve been extremely useful for testing and feel great in-game. Items placed in action bar slots are removed from the player’s backpack, effectively freeing up inventory space. Players can also rearrange or swap items between the inventory and action bar at will.
Hot bar functionality is smooth, modular, and already feels like a core quality-of-life feature. It's another solid step forward in making the gameplay loop more fluid and tactical.
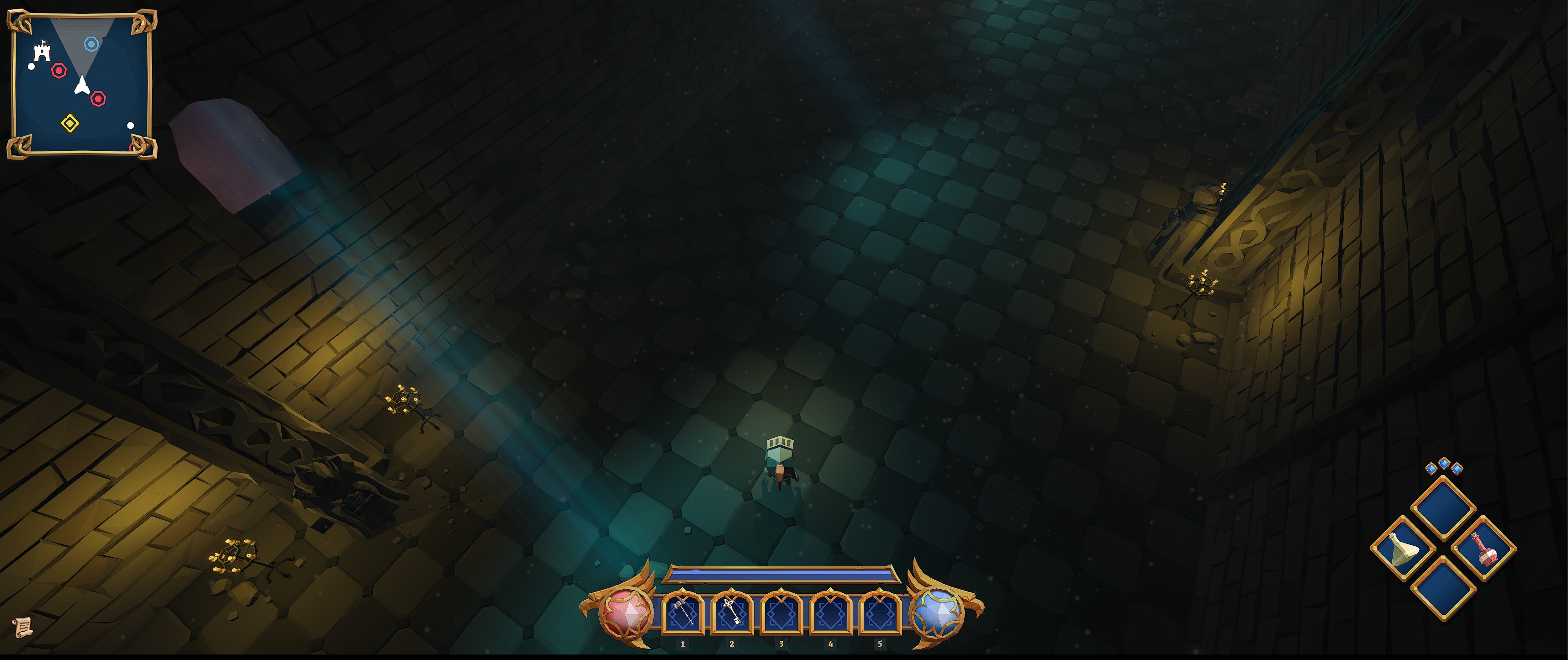
Player Death & Respawn
The player death system just got a major upgrade! When a player dies, they now trigger a proper death animation followed by a dramatic “You Died!” game over screen — setting the tone before they return to action.
Instead of ending the session, the game now freezes the player in their death pose until the respawn logic kicks in. Once the timer ends, the player respawns fully healed and re-energized, ready to face danger again.
Currently, there’s just one respawn point, but I’m planning to implement sanctuary-based respawns in the near future, allowing players to return to the last visited safe zone. This system feels way more immersive now and opens the door for future enhancements like revive mechanics or corpse runs.
Closing
Some of these systems really tested my patience — especially networking inventory data and syncing interaction logic for all players. But I learned a ton and now I have a reusable framework for both items and traps.
For the next devlog, I’ll be diving deeper into trap logic, introducing new types and interactions. I’ll also be kicking off the next two player stats: Strength and Dexterity, which will start influencing health pools and stamina regeneration respectively. On top of that, I’ll begin designing new dungeon rooms for the first map and start working on custom ambient music and sound effects using FL Studio.
Every piece that’s added makes the world feel more alive — and I’m excited to see it all come together.
Until next time...
Torches & Treasures
Status | In development |
Author | GhostdogStudio |
Genre | Adventure, Role Playing |
Tags | dd, dungeon, dungeoncrawler, indiedev, Tabletop, Top-Down, torchestreasures, tt, Unity |
More posts
- Update #6 Objectives, Currency and New Puzzle6 days ago
- Update #4 Health, Damage & Traps19 days ago
- Update #3 Door & Key System24 days ago
- Update #2 Continued Player Movement & Map29 days ago
- First Log!38 days ago
Leave a comment
Log in with itch.io to leave a comment.